SweepWidget API Documentation
The SweepWidget API allows you to read and write data to your giveaways. If you need any specific functionality that isn’t currently available, feel free to contact us.
How to find your API key?
First, you must get your API Key. Navigate to Integrations and click on API Access.
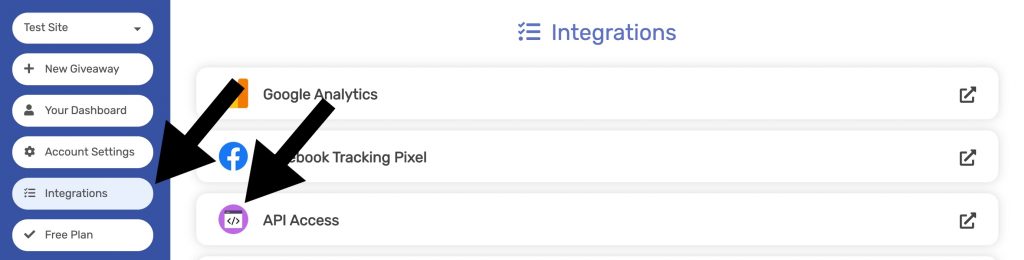
Then, copy your API Key.
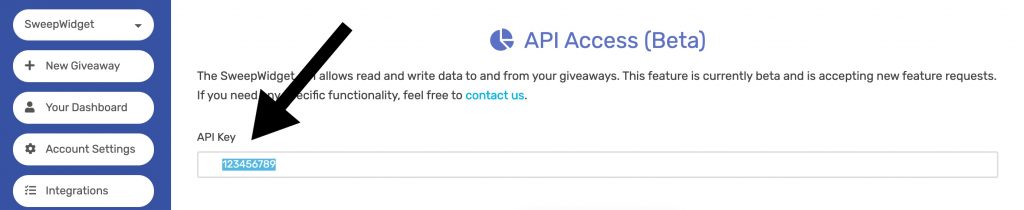
Accepted API Endpoints
The following API endpoints are accepted:
- List all unique users for a giveaway
- List all entries for a giveaway
- List all giveaways
- List all entries for a single user in a giveaway
- List all giveaways a user has entered
- Update whitelisted emails
- Add new entry method
- Add manual entries for a user
- Enter user into a giveaway
- Fetch referral link for a user
List all unique users for a giveaway
This method allows you to get each unique user that’s entered a giveaway.
It uses the following endpoint with the GET method.
https://sweepwidgetapi.com/sw_api/users
Parameters
The following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
competition_id | Integer | ID of your competition. | Yes |
page_start | Integer | The page you want to start on. JSON output returns 50 rows at a time. | Yes |
Example implementation
PHP example:
$curl = curl_init();
$url_get_lists = "https://sweepwidgetapi.com/sw_api/users?api_key=123456789&page_start=1&competition_id=123";
curl_setopt_array($curl, array(
CURLOPT_URL => $url_get_lists,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"Content-Type: application/json" // You must set the content-type to application/json
),
));
$response = json_decode(curl_exec($curl));
var_dump($response);
Example output
{
"data": [
{
"user_id": 123,
"user_name": "Test User",
"user_email": "[email protected]",
"birthday": "04-25-1994",
"entry_type": "Twitter Follow",
"timestamp": "2020-06-26 11:18:21"
"country": "United States"
}
]
}
List all entries for a giveaway
This method allows you to access all of the user entry information for a giveaway.
It uses the following endpoint with the GET method.
https://sweepwidgetapi.com/sw_api/entries
Parameters
The following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
competition_id | Integer | ID of your competition. | Yes |
page_start | Integer | The page you want to start on. JSON output returns 50 rows at a time. | Yes |
Example implementation
PHP example:
$curl = curl_init();
$url_get_lists = "https://sweepwidgetapi.com/sw_api/entries?api_key=123456789&page_start=1&competition_id=123";
curl_setopt_array($curl, array(
CURLOPT_URL => $url_get_lists,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"Content-Type: application/json" // You must set the content-type to application/json
),
));
$response = json_decode(curl_exec($curl));
var_dump($response);
Example output
{
"data": [
{
"user_id": 123,
"user_name": "Test User",
"user_email": "[email protected]",
"birthday": "04-25-1994",
"entry_type": "Twitter Follow",
"action": "Follow @SweepWidget On Twitter",
"value": "@MyTwitter",
"entry_amount": "5",
"timestamp": "2020-06-26 11:18:21"
"country": "United States"
}
]
}
List all giveaways
This method allows you to access basic information about all live, scheduled, and expired giveaways.
It uses the following endpoint with the GET method.
https://sweepwidgetapi.com/sw_api/giveaways
Parameters
The following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
type | String | Accepts any of the 3 values: live, scheduled, expired | Yes |
page_start | Integer | The page you want to start on. JSON output returns 50 rows at a time. | Yes |
Example implementation
PHP example:
$curl = curl_init();
$url_get_lists = "https://sweepwidgetapi.com/sw_api/giveaways?api_key=123456789&type=live&page_start=1";
curl_setopt_array($curl, array(
CURLOPT_URL => $url_get_lists,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"Content-Type: application/json" // You must set the content-type to application/json
),
));
$response = json_decode(curl_exec($curl));
var_dump($response);
Example output
{
"data": [
{
"competition_id": 123,
"competition_url": "ahej14f9",
"type": "Live",
"title": "My Giveaway Title",
"description": "This is the description of my awesome giveaway!",
"rules": "US, CA, 18+",
"start_time": "2020-07-28 00:00:00",
"end_time": "2020-08-28 00:00:00",
"time_zone": "America/Chicago",
"number_of_winners": "5"
"image_loc": "https://sweepwidget.com/images/my-image.jpg",
"giveaway_embed_code": "PGRpdiBpZD0iNjEyODctMHlwbXc3MTkiIGNsYXNzPSJzd19jb250YWluZXIiPjwvZGl2PjxzY3JpcHQgdHlwZT0idGVa4dC9qYXZhc2yaXB0IiBzcmM9Imh0dHBzOi8vc3dlZXB3aWRnZXQuY29tL3cvai93X2luaXQuanMiPjwvc2NyaXB0Pg" // <-- You have to base64_decode() this value!
}
]
}
IMPORTANT! The giveaway_embed_code is outputted in base 64 encoded format (because it’s HTML). So, you have have to base 64 decode this value to get the HTML value. e.g. in PHP, the function would be base64_decode($value);
List all entries for a single user in a giveaway
This method allows you to list all of the entries a user has completed in a single giveaway.
It uses the following endpoint with the GET method.
https://sweepwidgetapi.com/sw_api/user-entries
Parameters
The following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
competition_id
|
Integer | ID of your competition. | Yes |
user_email | string | This is the email address of the user who entered. | Yes |
Example implementation
PHP example:
<?php
$curl = curl_init();
$url = "https://sweepwidgetapi.com/sw_api/user-entries?api_key=12345&competition_id=123&[email protected]";
curl_setopt_array($curl, array(
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"Content-Type: application/json" // You must set the content-type to application/json
),
));
$response = json_decode(curl_exec($curl));
var_dump($response);
Example output
array(4) {
[0]=>
object(stdClass)#2 (5) {
["entry_type"]=>
string(12) "User Details"
["action"]=>
string(5) "Login"
["value"]=>
string(13) "Auto Verified"
["entry_amount"]=>
int(1)
["timestamp"]=>
string(19) "2024-02-29 15:13:51"
}
[1]=>
object(stdClass)#3 (5) {
["entry_type"]=>
string(19) "Facebook Page Visit"
["action"]=>
string(4) "NULL"
["value"]=>
string(13) "Auto Verified"
["entry_amount"]=>
int(1)
["timestamp"]=>
string(19) "2024-03-01 19:44:52"
}
[2]=>
object(stdClass)#4 (5) {
["entry_type"]=>
string(16) "Instagram Follow"
["action"]=>
string(4) "NULL"
["value"]=>
string(4) "Test"
["entry_amount"]=>
int(1)
["timestamp"]=>
string(19) "2024-03-01 19:44:58"
}
[3]=>
object(stdClass)#5 (5) {
["entry_type"]=>
string(15) "Email Subscribe"
["action"]=>
string(4) "NULL"
["value"]=>
string(13) "Auto Verified"
["entry_amount"]=>
int(1)
["timestamp"]=>
string(19) "2024-03-04 19:07:05"
}
List all giveaways a user has entered
This method allows you to list every giveaway a user has entered. It returns the competition_id for every giveaway they entered that you created. Note: it won’t return giveaways they entered that were created by other hosts.
It uses the following endpoint with the GET method.
https://sweepwidgetapi.com/sw_api/user-entered-giveaways
Parameters
The following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
search_value | String | Either the user_id or user_email of the user. | Either user_id or user_email value is required. |
search_key | String |
Accepted values: user_id OR user_email You must specify if you’re searching for a user by their id or email address. |
Either user_id or user_email is required. |
Example implementation
PHP example:
$curl = curl_init();
// Example searching by the user's id
$url_get_lists = "https://sweepwidgetapi.com/sw_api/user-entered-giveaways?api_key=123456789&search_value=123&search_key=user_id";
// Example searching by the user's email address
$url_get_lists = "https://sweepwidgetapi.com/sw_api/user-entered-giveaways?api_key=123456789&[email protected]&search_key=user_email";
curl_setopt_array($curl, array(
CURLOPT_URL => $url_get_lists,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"Content-Type: application/json" // You must set the content-type to application/json
),
));
$response = json_decode(curl_exec($curl));
var_dump($response);
Example output
{
"competition_id": [
{
[0]=>
string(5) "20234"
[1]=>
string(5) "20165"
[2]=>
string(5) "20137"
[3]=>
string(5) "20108"
]
}
}
Update whitelisted emails
This method allows you update the whitelisted emails for a single competition. You can optionally update the globally whitelisted emails for all competitions.
It uses the following endpoint with the POST method.
https://sweepwidgetapi.com/sw_api/white-list-emails
Parameters
All of the following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
global | Boolean | Specify if this is for a single giveaway or ALL giveaways in your account. Accepted values: 1 or 0. | Yes |
competition_id | Integer | ID of your competition. | Required only if global is set to 0. |
whitelisted_emails | Array | Specify which emails are allowed to enter. | Yes |
Example implementation
PHP example:
$post = [
'api_key' => '123456789',
'global' => 0,
'competition_id' => '123',
'whitelisted_emails' => array('[email protected]', '[email protected]', '[email protected]')
];
$post_string = http_build_query($post);
$ch = curl_init('https://sweepwidgetapi.com/sw_api/white-list-emails');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $post_string);
$response = curl_exec($ch);
curl_close($ch);
var_dump($response);
Add new entry method
This method allows you to add a new entry method to an existing giveaway.
It uses the following endpoint with the POST method.
https://sweepwidgetapi.com/sw_api/create-entry-method
Parameters
All of the following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
competition_id | Integer | ID of your competition. | Yes |
entry_order
|
Integer | What order the entry method appears. | Yes |
entry_method_type
|
String | Type of entry method. The following entry method types are available: Available entry method types. | Yes |
entry_method_handle
|
String | Title for the entry method on the outside (the title before the entrant toggles the entry method open). | Yes |
mandatory
|
Integer | Whether not it’s a required entry method. | Yes |
entries_worth
|
Integer | How many entries the entry method is worth. | Yes |
entry_link
|
String | This is either the profile handle or link depending on which one is required. Example: Instagram only requires the profile handle while Facebook requires the full link. You can reference the entry method while creating a giveaway to see which one is required. | Yes |
input_header
|
String | Header string after the entrant toggles open the entry method. | Yes |
additional_instructions
|
String | Optional additional instructions (Most entry methods don’t use this). | No |
widget_display
|
Integer | 1 = circle icon, 2 = square look (circle icon is the default). | No |
icon_color
|
String | Hex color code for icon e.g. #3b5998 | No |
require_verification
|
Integer | If you require the user to verify the username they performed the entry method with e.g. user must enter their Instagram username after following you. | No |
language
|
String | 2 character language code. Default is english: en | No |
Available entry method types
Use this values in the entry_method_type parameter.
- attend_eventbrite_event
- attend_eventbrite_venue
- bloglovin_follow
- discord_join_server
- disqus_comment
- ebay_follow
- etsy_follow
- etsy_item
- facebook_group_visit
- facebook_page_visit
- facebook_post_visits
- feedburner
- feedpress
- instagram_follow
- instagram_like_post
- instagram_repost
- instagram_submit_post
- instagram_view_post
- instagram_visit_profile
- linkedin_follow
- linkedin_share
- mixer_follow
- myspace_follow
- patreon_page_visit
- pinterest_follow_board
- pinterest_follow_user
- pinterest_repin_pin
- pinterest_submit_board
- pinterest_submit_pin
- pinterest_visit_page
- pinterest_visit_pin
- reddit_subscribe
- rss
- snapchat_follow
- soundcloud_follow
- soundcloud_like_song
- soundcloud_listen_to_song
- soundcloud_repost_song
- soundcloud_submit_song
- spotify_follow
- spotify_listen_to_song
- steam_join_group
- telegram_join_channel
- tiktok_comment
- tiktok_follow
- tiktok_like
- tiktok_share
- tiktok_visit
- tiktok_watch_video
- tumblr_follow
- tumblr_like_post
- twitch_follow
- twitch_subscribe
- twitter_follow
- twitter_retweet
- twitter_tweet
- youtube_channel_subscribe
- youtube_comment
- youtube_like_video
- youtube_submit_video
- youtube_visit_channel
- youtube_watch
Example implementation
PHP example:
$post = [
'api_key' => '123456789',
'competition_id' => '123',
'entry_order' => '5',
'entry_method_type' => 'facebook_page_visit',
'entry_method_handle' => 'Visit Us On Facebook',
'mandatory' => '0',
'entries_worth' => '10',
'entry_link' => 'https://facebook.com/SweepWidget',
'input_header' => 'Visit and follow SweepWidgetTEST on Facebook by manually clicking on the button below.',
'additional_instructions' => '',
'widget_display' => '1',
'icon_color' => '#3b5998',
'require_verification' => '1',
'language' => 'en'
];
$post_string = http_build_query($post);
$ch = curl_init('https://sweepwidgetapi.com/sw_api/create-entry-method');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $post_string);
$response = curl_exec($ch);
curl_close($ch);
var_dump($response);
Add manual entries for a user
This method allows you to add manual entries for a user. The user must already be entered into your giveaway to add entries. For example, if there is a user who as already entered your contest under the email [email protected], you can manually add 1-10,000 entries for this user.
It uses the following endpoint with the POST method.
https://sweepwidget.com/sw_api/add-manual-entries
Parameters
All of the following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
competition_id | Integer | ID of your competition. | Yes |
entry_amount
|
Integer | How many entries you are giving to this user. | Yes |
user_email
|
String | The email address of the user. Note: the user must already be entered into your contest. | Yes |
Example implementation
PHP example:
$post = [
'api_key' => '123456789',
'competition_id' => '123',
'entry_amount' => '1',
'user_email' => '[email protected]'
];
$post_string = http_build_query($post);
$ch = curl_init('https://sweepwidget.com/sw_api/add-manual-entries');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $post_string);
$response = curl_exec($ch);
curl_close($ch);
var_dump($response);
Enter user into a giveaway
This method allows you to enter a user into your giveaway using the API.
It uses the following endpoint with the POST method.
https://sweepwidgetapi.com/sw_api/new-entry
Parameters
All of the following parameters are accepted
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
competition_id | Integer | ID of your competition. | Yes |
user_email
|
String | Email of the user | Yes |
user_name
|
String | Name of user | Yes |
entry_amount
|
Integer | How many entries is this worth? | Yes |
giveaway_link
|
String | Full URL to where the giveaway is located. Example (https://yoursite.com/path-to-your-giveaway). Note: you must include the website protocol i.e. http:// or https:// | Yes |
sw_share
|
String |
This value is the share hash from the share link. You must fetch this value from the share link on your website. The GET variable will be sw-share. Example: https://yourwebsite.com/path-to-your-giveaway?sw-share=12345-asfdjhwe. You must fetch the value of sw-share which is equal to 12345-asfdjhwe. You must then pass it to sw_share parameter. The API will know who referred this user. You must conditionally pass this parameter only if the share hash is set in your URL. Note: in order for this feature to work, you must create a refer a friend entry method on the giveaway you’re using the API for. |
No |
Example implementation
PHP example:
$curl = curl_init();
$post = [
'api_key' => '12345',
'competition_id' => '123',
'user_email' => '[email protected]',
'user_name' => 'Test User',
'entry_amount' => '1',
'giveaway_link' => 'https://example.com/path-to-my-giveaway', // This should be the link where your giveaway is hosted e.g. on your website or the hosted landing.
//'sw_share' => '8u84os-12345' // This paremter is only if the referral link is set i.e. ?sw-share=8u84os-12. You must conditionally pass this parameter on your end.
];
$post_string = http_build_query($post);
$ch = curl_init('https://sweepwidgetapi.com/sw_api/new-entry');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $post_string);
$response = curl_exec($ch);
curl_close($ch);
var_dump($response);
Fetch referral link for a user
This method allows you to fetch a user’s referral link for any giveaway.
It uses the following endpoint with the GET method.
https://sweepwidgetapi.com/sw_api/fetch-user-referral-link
Parameters
All of the following parameters are accepted.
Parameter | Format | Description | Required |
---|---|---|---|
api_key | String | API developer key. | Yes |
competition_id | Integer | ID of your competition. | Yes |
user_email
|
String | Email of the user | Yes |
Example implementation
PHP example:
$curl = curl_init();
$url_get_lists = "https://sweepwidgetapi.com/sw_api/fetch-user-referral-link?api_key=12345&competition_id=123&[email protected]";
curl_setopt_array($curl, array(
CURLOPT_URL => $url_get_lists,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"Content-Type: application/json" // You must set the content-type to application/json
),
));
$response = json_decode(curl_exec($curl));
var_dump($response);